Frontend Architecture: The Road to Reusable, Scalable, and Maintainable Components
Yacine Ouardi
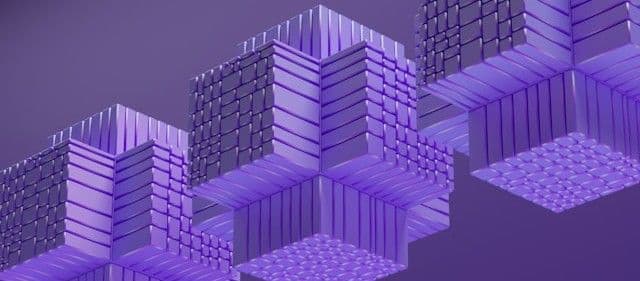
When I first started building frontend applications, I thought writing good code meant keeping everything DRY, using the latest libraries, and following whatever architecture pattern was trending on Twitter. Fast forward a few years, and I’ve realized that frontend architecture isn’t about trends or perfection. It’s about building reusable, scalable, and maintainable components that can survive the inevitable growth and complexity of real-world applications.
In this article, I’ll share the principles I’ve learned along the way—framework-agnostic at their core, but with examples from React and Next.js, the tools I use every day.
Why Does Frontend Architecture Matter?
At first glance, it’s easy to dismiss architecture as something only backend engineers or large teams need to worry about. But even a solo developer (like me, building side projects while working full-time) can feel the pain of poor architecture: tangled dependencies, brittle components, duplicated logic, and a UI that’s hard to update without breaking something.
Good frontend architecture isn’t just for scalability in terms of users—it’s about scalability in development: the ability to ship new features, onboard teammates, or refactor safely without pulling your hair out.
The Double-Edged Sword of Reusable Components
We all love reusable components—until they become so generic that they’re impossible to extend or debug. I’ve fallen into the trap of abstracting too early, creating a single Button
component with 20 props to cover every possible use case. Sure, it reduced duplication, but it also made every button harder to use and test.
📝 Lesson learned: Reusability should emerge from patterns, not be forced upfront. Start specific, then abstract when duplication and patterns naturally appear.
In React/Next.js, this might look like starting with:
jsx
export function PrimaryButton({ children, onClick }) {
return <button
className="bg-blue-600 text-white px-4 py-2 rounded"
onClick={onClick}>{children}
</button>;
}
And only later creating a generic <Button>
once multiple button types actually need it.
Scaling Components: Composition Over Inheritance
One of the best lessons React taught me is the power of composition. Instead of stuffing components with props and configuration, I try to let the children prop and composition handle extensibility.
Example:
jsx
<Card>
<CardHeader title="Welcome!" />
<CardContent>Here’s some important content.</CardContent>
<CardFooter>Footer info here.</CardFooter>
</Card>
This pattern keeps each piece responsible for its own part, and assembling them feels natural. It’s easier to scale a component tree this way than relying on deep prop chains or inheritance-based overrides.
Maintainability: Naming, Structure, and Co-Location
A reusable, scalable component is useless if nobody knows where to find it or how to use it.
Here’s what’s worked for me:
✅ Co-locate styles, tests, and component logic in the same folder. If I build a Card
component, everything related to Card
lives in /components/Card/
: Card.jsx
, Card.module.css
, Card.test.jsx
, etc.
✅ Consistent naming conventions. I follow PascalCase for components, camelCase for functions/variables. Sounds basic, but consistency is 80% of clarity.
✅ Flat folder structures over deep nesting (until nesting is unavoidable). I’ve seen codebases with /components/atoms/forms/inputs/textInput/TextInput.jsx
—good luck navigating that.
Avoiding Common Pitfalls
I wish someone had warned me about these earlier:
🚩 Over-abstraction: If you build an abstraction for everything, you’ll end up spending more time writing wrappers than features.
🚩 Premature optimization: You don’t need a global state management library or microfrontend setup on day one. Optimize for today’s complexity, not hypothetical future problems.
🚩 Tight coupling between UI and business logic: Keep your components focused on rendering and interaction. Move data fetching, transformations, and business rules into hooks or services.
A Real-World Example: Lexonate’s Dashboard
When I built the dashboard for Lexonate, my side project for managing internalization, I faced this exact challenge. I started with a simple table view to list entries. As features grew—pagination, filters, inline editing—I realized the need for reusable table components.
But instead of jumping straight to a generic <Table>
with 20 props, I broke it into composable parts: <Table>
, <TableHeader>
, <TableBody>
, <TableRow>
, <TableCell>
. Each part was a thin wrapper with specific responsibility. This let me reuse pieces across different contexts without overcomplicating the API.
That experience reinforced something: scalable frontend architecture is more about thinking in small, composable units than building big generic ones.
Final Thoughts: Aim for Pragmatic, Not Perfect
Frontend architecture isn’t about chasing perfection. It’s about finding structures and patterns that make your app easier to grow, debug, and maintain over time.
✅ Don’t abstract too early.
✅ Favor composition.
✅ Keep things close and consistent.
✅ Avoid over-engineering for problems you don’t have yet.
And remember: no architecture decision is set in stone. You can always evolve as your app grows. I’m still learning and refining every project I work on—and if you want to see these principles in action, feel free to browse my portfolio or reach out.
Thanks for reading! If this resonated with you, I’d love to hear how you’re tackling frontend architecture in your projects.