When to Stop Refactoring: How Much Is Too Much?
Yacine Ouardi
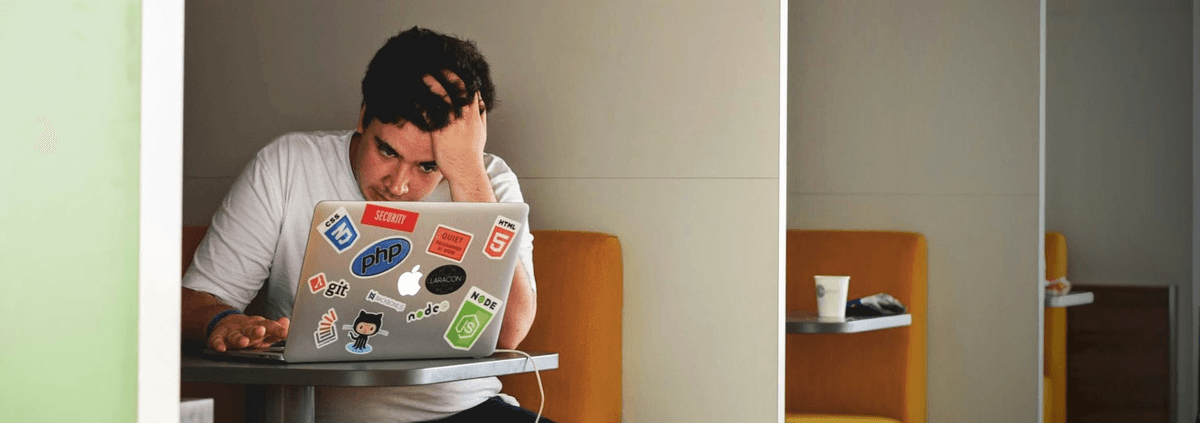
I’m gonna be honest—this is something I’ve struggled with a lot.
You know that feeling when your code works, but you just know you can clean it up? You tell yourself, “Ah, I’ll just tidy this real quick.” Fast forward: it’s hours later, and you’ve basically rewritten the whole thing… for no real reason.
I’ve fallen into that trap so many times, and it took me a while (and some hard lessons) to figure out where the line is.
Why We Get Obsessed With Refactoring
We all want our code to be clean and beautiful, right? It’s almost like a point of pride. Plus, good refactoring makes things easier to read and maintain.
But here’s the thing I learned the hard way: there’s a fine line between making your code better and just... endlessly tweaking it because you can.
Early in my career, I thought refactoring was always the right move. Like, if something could be cleaner, then I should make it cleaner. But the problem? That mindset can easily spiral. I’d catch myself refactoring tiny components that no one else even touched—wasting time that could’ve been spent shipping actual features.
A Personal Screw-Up
One moment really sticks in my head. We were working on a deadline, and I had this component that was working fine but felt messy to me. I thought, “I’ll refactor it quickly—no big deal.”
Big mistake.
That “quick” refactor turned into two full days of me overcomplicating everything. By the end, sure, the code was “cleaner”… but I had broken stuff that wasn’t broken before. I missed the deadline, frustrated my team, and honestly? The new version wasn’t even that much better.
That was the wake-up call I needed.
How I Decide Now
I’ve learned to ask myself a few questions before I dive into refactoring:
- Is this solving an actual problem?
Not just my itch to clean it up—does it fix confusion or bugs? - Will it save time in the long run?
Refactoring is only worth it if it prevents real headaches down the line. - Is this the right time?
If we’re in crunch mode or close to a release, I now force myself to hold off.
This simple gut check has saved me (and my team) a ton of unnecessary work.
A Small Example
This might sound familiar if you’ve worked with React:
jsx
function UserProfile({ user, isAdmin }) {
return (
<div>
<h2>{user.name}</h2>
{isAdmin && (
<div>
<button onClick={() => promoteUser(user.id)}>Promote</button>
<button onClick={() => deleteUser(user.id)}>Delete</button>
</div>
)}
</div>
);
}
It works fine. But later we needed to add more admin actions, and it will start to feel bloated. So instead of cramming everything into UserProfile
, we should split it out like this:
jsx
function UserProfile({ user, isAdmin }) {
return (
<div>
<h2>{user.name}</h2>
{isAdmin && <AdminActions userId={user.id} />}
</div>
);
}
function AdminActions({ userId }) {
return (
<div>
<button onClick={() => promoteUser(userId)}>Promote</button>
<button onClick={() => deleteUser(userId)}>Delete</button>
{/* More admin buttons could go here */}
</div>
);
}
That was a good refactor: clear purpose, easier to extend, and didn’t waste time.
The Perfectionism Trap
The biggest lesson? Not every piece of code has to be a work of art. I used to believe that if something wasn’t “perfect,” it wasn’t good enough. But that’s just not practical—especially when you’re working in a team and shipping real products.
Good enough really is good enough most of the time.
Final Thoughts
If you’re like me and have that urge to keep polishing your code endlessly, take a breath and ask yourself:
Am I actually solving a problem, or am I just avoiding the next task?
Learning when to stop is just as important as knowing how to refactor in the first place.